Back to blog
What Is Boilerplate Code? How to Use It Effectively
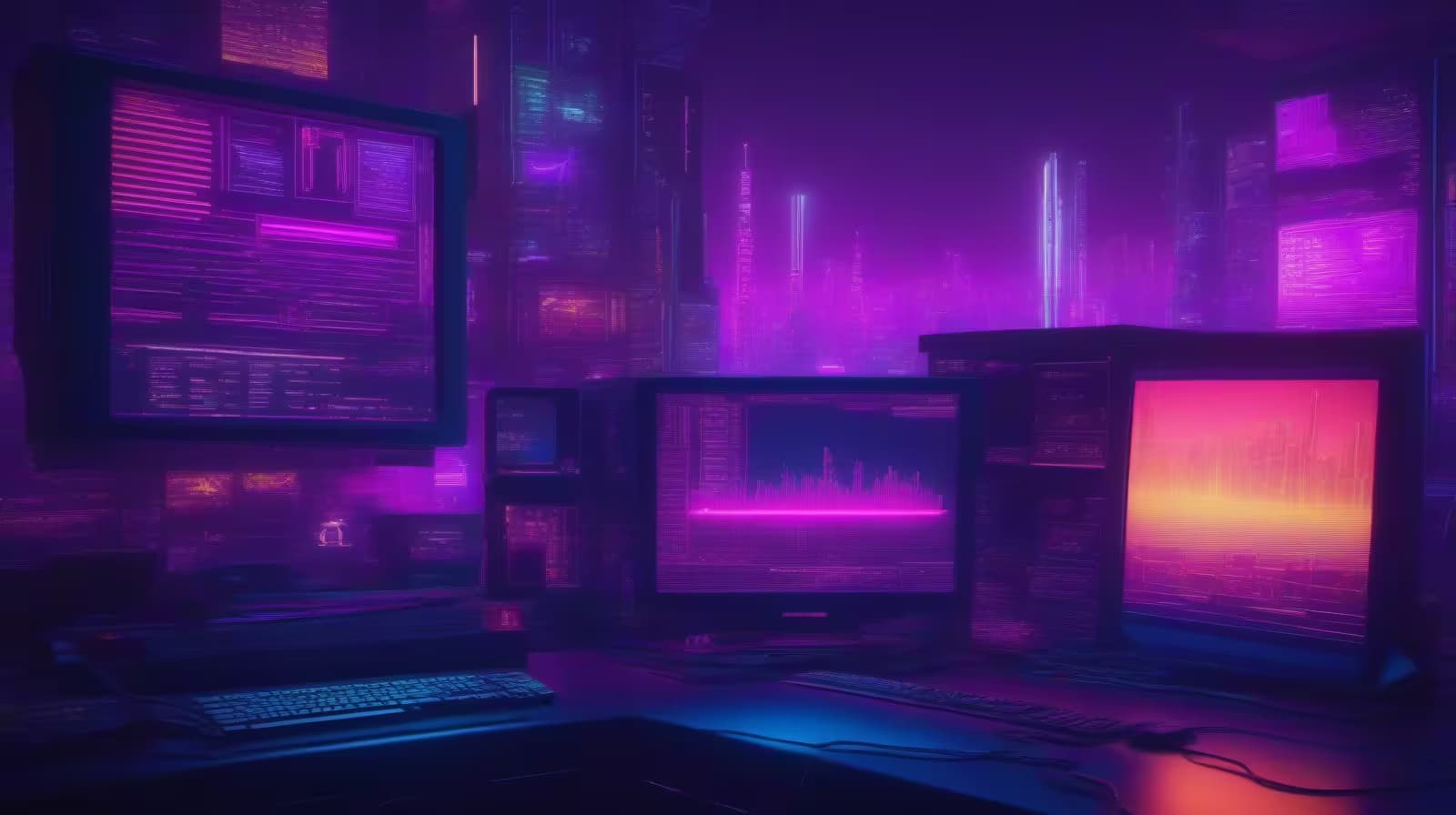
Table of content
Boilerplate code, or template code, is a term used to refer to code that is repeated in various blocks throughout your project or even in different projects.
Boilerplate code is code that remains the same in all the places it is used, which leads to questions like:
- If the code is the same, why not abstract it and reuse it?
- If I slightly adapt the code, is it no longer boilerplate?
- Is using boilerplate code a good practice?
All of these questions are valid, and I will try to answer them throughout this article. Before we can answer the above questions, it's important that we have a clear understanding of what boilerplate code is.
Examples of Boilerplate
Boilerplate code can come in any size and shape, from a few characters to an entire project with different programming languages and technologies. The important thing is that it's code that we, as developers, reuse.
HTML Boilerplate
Probably the most common boilerplate is the HTML one. Surely most developers have written the following code at some point, and perhaps more than once. Without the following code snippet, we couldn't create a web page.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body></body>
</html>
Looking at the above code, some might think that it's not so bad and could be written quickly. But what if we make a slight mistake? For example, we forget to add the lang attribute in the html tag, or we make a typographical error with with instead of width, or maybe we forget to include the meta tag to control the viewport behavior. The results may not be as expected. This would lead to wasted time debugging and correcting errors.
The importance of maintaining a standard in our code is high, and having to write the same code several times is a tedious task. Even more so if we have to ensure that the code is consistent.
You probably have a clearer idea of what boilerplate is with this example and start to see its importance. Now, what if I slightly modify my boilerplate code for different occasions? Is it no longer boilerplate? Is it a bad practice to modify it?
For example, we can agree that we don't always want our title tag to have the same value Document.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>My New and Amazing Web Application!</title>
</head>
<body></body>
</html>
The first code snippet I showed you is what we would call our boilerplate. It is immutable, and we should not modify it. On the other hand, this last code snippet is not boilerplate code itself. This second code snippet is the utilization of our boilerplate code adapted to our needs.
So, to answer the first question, yes, we can modify our boilerplate code, but we should not modify it directly; instead, we should modify it in its usage. Is it a bad practice to modify it? It depends. If when inserting our boilerplate, we constantly find ourselves modifying it to values that are the same over and over, then it's probably a bad practice, and we should extract a new boilerplate code from the modified code. On the other hand, if the boilerplate code we modify is highly circumstantial and does not repeat frequently, then it is definitely not a bad practice.
Let's continue expanding our understanding of boilerplate code with other examples.
CSS
All of us who write CSS daily can say that we write the following code snippet probably about a hundred times a day.
.class {
/* my styles */
}
But are these few characters considered boilerplate code? The answer is yes. This code snippet is boilerplate code. However, we could argue that it's not very useful. The time it would take to find the code snippet and insert it into our editor is longer than simply writing it, which distracts us.
As a general rule, if boilerplate code takes more time to insert than to write, it's better not to use it.
Now, let's write a more useful CSS snippet.
& > *:not(:last-child) {
margin-right: 1rem;
}
Before CSS blessed us with the gap property in grid and flexbox, this code snippet was quite useful for spacing all child elements of a parent element without adding spacing after the last child.
I think we can agree that it wasn't a selector we liked to remember and write every time we needed it, so it was a good candidate for extraction as boilerplate code.
A more contemporary example, for instance:
button:has(svg) {
display: flex;
align-items: center;
justify-content: center;
gap: 0.5rem;
}
This selector is useful for styling buttons that contain an icon and is a good candidate for extraction as boilerplate code.
React
Now, what about React.js?
I'm using React in my example because it is currently the most popular JavaScript framework. But what I'm going to say applies to any framework.
Frontend frameworks are perfect for demonstrating the value of boilerplate code. A moderate-sized app likely contains hundreds of components, and all these components probably have a code snippet like the following.
import React from "react";
const Component = () => {
return <div>Component</div>;
};
export default Component;
Writing this hundreds of times is not only tedious but also a constant distraction before starting development. Additionally, each developer has their preferences for writing this snippet. For example:
Using traditional functions
import React from "react";
function Component() {
return <div>Component</div>;
}
export default Component;
Using named exports
import React from "react";
export function Component() {
return <div>Component</div>;
}
Since React 17, it's possible that you don't need to import React
export const Component = () => {
return <div>Component</div>;
};
The same result with different nuances. It makes sense to avoid having to write these code snippets every time you need a component and, instead, use boilerplate code to maintain a standard among all developers.
Java
As a final example, I'd like to talk about Java. Java is an object-oriented programming language, and it's quite common to have to write classes in Java. If you've programmed in Java, you know that its classes have quite a lengthy boilerplate code.
public class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
It's not surprising that new programming languages like Kotlin have emerged, aiming to reduce the boilerplate code of Java. If we use boilerplate code, writing this would be less painful, as we wouldn't lose as much time writing repetitive code.
Now, remember that these are just basic examples of boilerplate code. In your daily work, if you pay attention, you'll find that you can extract much more boilerplate code from your projects. In fact, I recommend doing so. It's a good practice. You save time and focus on what really matters.
What Boilerplate Tools Are Available?
The next question you might ask is: Okay, extracting boilerplate code and reusing it is an excellent idea. But where do I store it? How do I reuse it?
Your Code Editor
If you use VSCode, for example, you can use VSCode snippets to store and reuse your boilerplate code. Snippets are an excellent tool for saving and reusing code. VSCode recognizes the text you type and suggests snippets you can use.
Just click the gear icon at the bottom left of your editor and select the User Snippets option.
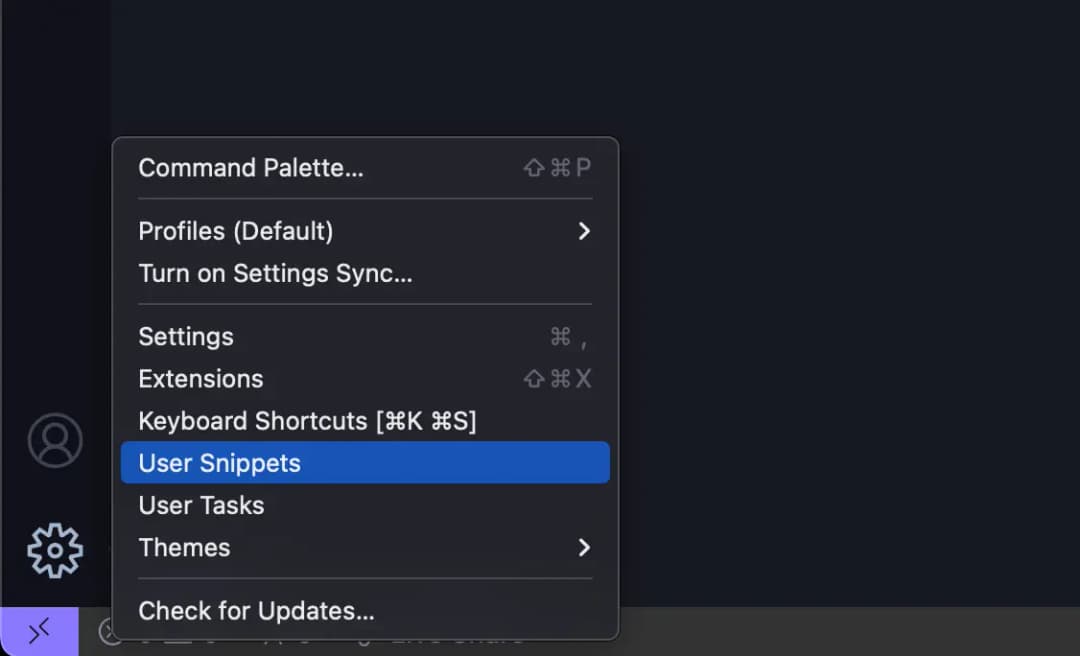
This will open a VSCode configuration file. In this file, you can add your snippets.
{
"My Snippets": {
"prefix": "my-snippet",
"body": ["My snippet"],
"description": "My snippet"
}
}
More info here: User Snippets
Extensions for one Developer
Whether they are extensions for your text editor or your web browser, there are some free options that will meet your boilerplate code needs if you work solo.
My number one recommendation is the one I used for years, Blueprint by teamchilla. This extension allows you to save boilerplate code and inject a value into specified places before inserting it. It was my favorite extension for years. However, unfortunately, it was not suitable for team collaboration and not flexible enough for my needs.
Extensions for Multiple Developers and Teams
If you work as part of a team and need a flexible tool that fits the workflow of your editor (VSCode), I recommend Archittect.
Disclaimer: I am one of the developers of Archittect. But I recommend it not because of that but because I spent years trying to find a tool for my team to share boilerplate code. I couldn't find a solution designed for this specific use case.
Archittect is a tool that allows you to save and share boilerplate code with your team. It not only helps you keep your boilerplate code organized and up to date but also helps you transform it into dynamic templates that adapt to your needs. I use it in my daily work, from small, easily forgotten lines of code to structures with multiple files and many dynamic values.
Here's the link to its extension in case you want to take a look: Archittect VSCode
Dynamic Boilerplate Code
You might have noticed that by using certain tools, you can turn your boilerplate code into dynamic code. If you're not already doing this, I recommend giving it a try. It will save you even more time than static boilerplate code.
Let's learn more about dynamic boilerplate code.
I like to think that there are three categories of dynamic boilerplate:
These definitions are not official; they are just a guide to help you better understand the concept.
Snippets
As simple as a single line or a block of code. Snippets are excellent for saving code snippets that you often forget or use frequently.
For example:
const [value, setValue] = useState(initialValue);
Using tools like Archittect, we can convert this snippet into a dynamic snippet. "Value" would become a variable that, when inserting the snippet, you would be prompted to replace with a value.
The important thing about snippets is that they can be a double-edged sword. Remember that if you type out the code snippet faster than it takes to insert it, you shouldn't use it. But if you have a tool optimized for this task, snippets become an excellent option for saving time and maintaining a code standard.
Templates
Templates, on the other hand, are more complex. They can range from a single block of code to several blocks. You can think of them as combining multiple snippets at once. This is my personal template for React components with TypeScript and TailwindCSS.
When inserting it, I replace Component with the name of my component, and it doesn't matter if I use camelCase or PascalCase in certain places. The template takes care of the transformation for me.
import React from "react";
import { twMerge } from "tailwind-merge";
type Props = {
className?: string;
};
const Component = (props: Props) => {
return <div className={twMerge("Component", className)}>Component</div>;
};
export default Component;
For example, if I want to create a Hero component, the result would be:
import React from "react";
import { twMerge } from "tailwind-merge";
type Props = {
className?: string;
};
const Hero = (props: Props) => {
return <div className={twMerge("Hero", className)}>Hero</div>;
};
export default Hero;
Scaffolds
Finally, scaffolds. When we start talking about more than one file, we are talking about scaffolds. Scaffolds are code templates that can contain multiple files and folders and can be as complex as you want.
For example, perhaps you like to place your unit tests near your components, or even your TypeScript types and interfaces. A scaffold can help you create a folder and file structure that fits your needs.
src/components/Component/
├── Component.test.tsx
├── Component.tsx
└── Component.types.ts
Or, for example, the file structure for your next project.
.env
.eslintrc
.prettierrc
.gitignore
src/
├── components
├── pages
├── styles
├── utils
└── ...
...
Insert as many dynamic variables as you want:
- Project name
- Library versions you'd like to use
- Color palette
- Configuration files
- Predefined tests
- The possibilities are limitless.
Conclusion
Writing boilerplate code can be a tedious task, but using the right tools, you can leverage it to save a lot of time and effort.
Automated boilerplate code will help you:
- Save time and money
- Avoid errors
- Maintain a code standard
Use it to your advantage and that of your team. Transform your static boilerplate into dynamic templates using tools like Archittect.
I hope this article has helped you understand what boilerplate code is and how you can use it to your advantage. If you have any questions or comments, feel free to reach out to me on Twitter at @LuisEduardoDev.